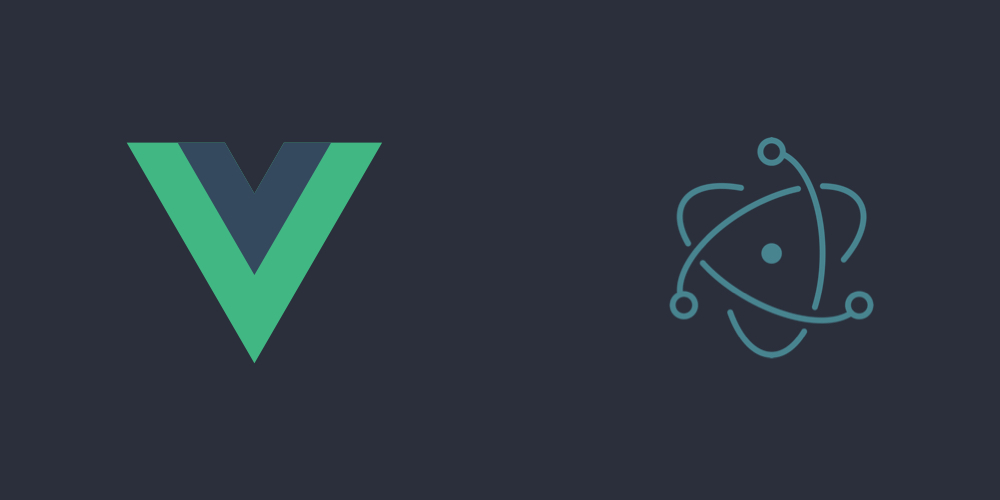
Electron is a framework for quickly developing cross-platform desktop applications. Together with Vue.js and Vuex an individual can be productive in a short period of time.
In a past project a client wanted a desktop app that supported multiple browser windows and all windows shared a state. That last point is a tricky one.
Electron Browser Windows are basically the same as a Chrome Browser Window since Electron is built on top of Chromium. The windows cannot communicate directly with each other and any communication has to be done through the main process.
The structure of windows will be a master/slave architecture. The main window will commit a mutation and the secondary window will follow suit. The main process will receive messages from the main window renderer and relay them to the desired target. ipcRenderer module will allow us to send a message from the windows to the main process. ipcMain module will allow us to listen to any messages sent by the main window.
The main process will listen for the vuex-mutation
message and then relay that information to the secondary window:
|
|
Any time the Vuex module commits a mutation we will send that data to the main process that will dispatch to all the other windows. Luckily Vuex supports a plugin architecture that allows us to subscribe to any mutations.
Lets create the plugin:
|
|
Add plugin to the vuex constructor:
|
|
The slave window needs to listen for vuex-scoreboard-mutation
messages from the main process and then relays that data to the vuex action. In the slave window add:
|
|
That’s it (more or less). Depending on your needs this could be most of the logic you require to get it working. You could go a step further and split your vuex code into modules, which is what I needed to do.
I actually needed a mostly synced state. You might also need to know if the current Vue instance is in the master or slave instance and that is where the states would differ.
Other things that could be added in order to make the windows state as desired or seamless as possible:
- Full state load when secondary window is created.
- Ignore unwated mutations in secondary window.
- Load from local storage.
Loading Comments